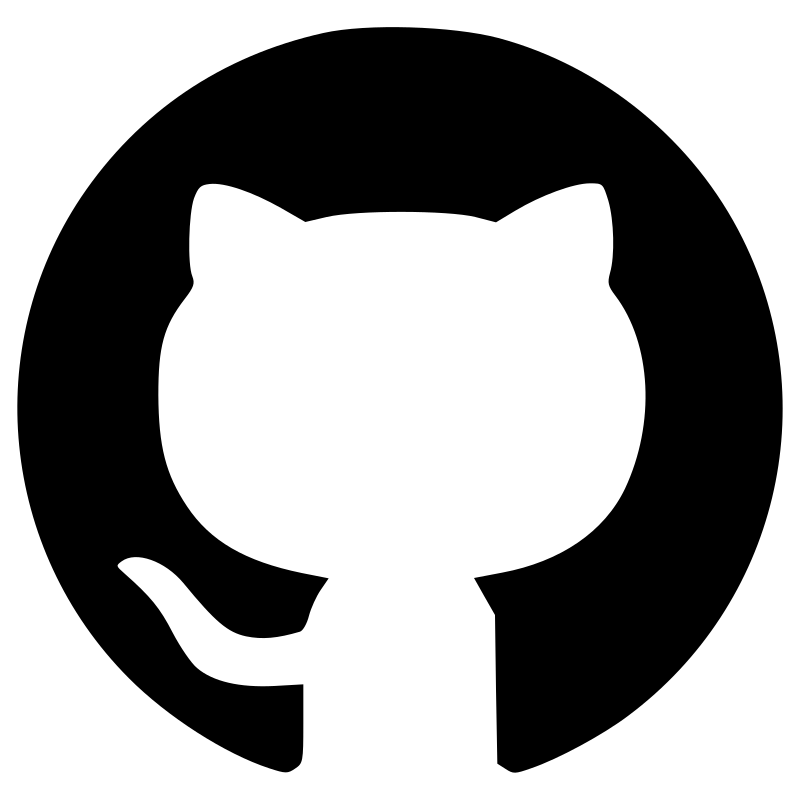
JavaScript (JS) is a lightweight interpreted (or just-in-time compiled) programming language with first-class functions. While it is most well-known as the scripting language for Web pages, many non-browser environments also use it, such as Node.js, Apache CouchDB and Adobe Acrobat. JavaScript is a prototype-based, multi-paradigm, single-threaded, dynamic language, supporting object-oriented, imperative, and declarative (e.g. functional programming) styles.
JavaScript is one of the most popular modern web technologies! As your JavaScript skills grow, your websites will enter a new dimension of power and creativity.
However, getting comfortable with JavaScript is more challenging than getting comfortable with HTML and CSS. You may have to start small, and progress gradually. To begin, let's examine how to add JavaScript to your page for creating a Hello world! example. (Hello world! is the standard for introductory programming examples.)
const myHeading = document.querySelector("h1");
myHeading.textContent = "Hello world!";
The heading text changed to Hello world! using JavaScript. You did this by using a function called querySelector() to grab a reference to your heading, and then store it in a variable called myHeading. This is similar to what we did using CSS selectors. When you want to do something to an element, you need to select it first.
Following that, the code set the value of the myHeading variable's textContent property (which represents the content of the heading) to Hello world!
A variable is a container for a value, like a number we might use in a sum, or a string that we might use as part of a sentence.
Declare a Variable:
let myName;
let myAge;
Initialize the Variable
myName = "Chris";
myAge = 37;
You can declare and initialize a variable at the same time, like this:
let myDog = "Rover";
You'll probably also see a different way to declare variables, using the var keyword:
var myName;
var myAge;
Back when JavaScript was first created, this was the only way to declare variables. The design of var is confusing and error-prone. So let was created in modern versions of JavaScript, a new keyword for creating variables that works somewhat differently to var, fixing its issues in the process.
A conditional statement is a set of commands that executes if a specified condition is true. JavaScript supports two conditional statements: if...else and switch.
An if statement looks like this:
if (condition) {
statement1;
}else {
statement2;
}
Here, the condition can be any expression that evaluates to true or false. (See Boolean for an explanation of what evaluates to true and false.)
If condition evaluates to true, statement_1 is executed. Otherwise, statement_2 is executed. statement_1 and statement_2 can be any statement, including further nested if statements.
Loops offer a quick and easy way to do something repeatedly.
These are some types of loops
- While Loops
- for Loops
- forEach Loops
- do-while Loops
A for loop repeats until a specified condition evaluates to false. The JavaScript for loop is similar to the Java and C for loop.
for (initialization; condition; afterthought)
statement
The do...while statement repeats until a specified condition evaluates to false.
do
statement
while (condition);
statement is always executed once before the condition is checked. (To execute multiple statements, use a block statement ({ }) to group those statements.)
A while statement executes its statements as long as a specified condition evaluates to true. A while statement looks as follows:
while (condition)
statement
All content in this website is from MDN Web Docs.
Find the references per topics below: